Code 2
Data and Variables
Learning Objectives:
In this module, you will...
- Learn about data and different data types
- Learn how to use variables and databases
- Learn to organize data into lists
- Create a to-do list app that connects to a database
This unit contains a lot of information, and it is okay if you don’t understand it all right now. The best way to understand coding concepts is by working through the Code Challenges to see them in action! You can always refer back to this unit anytime you get stuck building your final app.
Data
At some point in your life, you’ve probably heard the word data. Data can mean a lot of things, but in computer science, data is the information that your app needs in order to work. To better understand this, let’s think about some apps and some of the information that they need in order to work.
This social app can message and call contacts. The data it needs is:
- Your username
- Your friends' usernames
- Messages you want to send
- The time
- Your location
Angry Birds
In this game app you shoot birds at pigs to defeat them. The data it needs is:
- Your score
- How many points each object is worth
- What levels you've completed
- How many birds you get in each level
Slideshow Challenge App
This is the app you made in the Code 1 Challenge. The data it needs is:
- Your favorite images
The programmer needs to set up ways for the app to get all the information it needs. Remember, computers can’t do anything on their own without instructions. Here are a few ways that apps in App Inventor can get data to use:
- The programmer put the information in:
- In your slideshow app, you (the programmer) entered in your favorite images.
- In Angry Birds, the programmer entered in how many birds the player gets on each level.
- The user enters the information:
- In WhatsApp, the user needs to tell the app what she wants to send and who she wants to send it to.
- The app connects to another place to get information:
- WhatsApp connects to your phone to get the time so it knows what time you sent a message.
Sometimes an app will save information the user put in. For example, WhatsApp saves message history which allows you to see the last message you sent to a friend. To do this in, the programmer uses a database, which you will learn about in this lesson. You will learn more about how to connect to other places to get information later.
Thought Exercise
If you were using an app that helps you find the nearest grocery store, what data do you think the app needs to have?
Data Types
As you saw in the section above, data can be a lot of things! To make it easier for computers to understand what to do with the data they are given, data is put into categories. This is known as a data type. Programming languages revolve around three major data types: numbers, strings, and booleans. App Inventor also lets you use more complex data types such as colors and images. You don’t need to do anything with data types you'll learn about in this section but it will be good to keep them in mind as you code more.
Numbers
A number data type is just what you think it is! In App Inventor, you can use numbers by clicking on going to Math in blocks.
You will use Math blocks whenever you need to use numbers. Here are some examples of when you might use a number data type in your app.
- Calculating a player’s score in a game
- Counting how many times a user has tried to log in
- Counting how many times you want your app to do something
- Checking to see if your user is a certain age
To learn more about all the things you can do with math blocks check out MIT’s website: Math blocks
Strings
A string data type is text. In App Inventor, you can create a string by going to Text in Blocks. Here are some the text blocks you can use.
You should use a string anytime you want to add a text field to your code. Here are some examples of when you might use a string data type in your app.
- Allowing the the user to enter a password or username
- Having the app speak words to the user
- Showing a message or an alert to the user
- Programming the app to open a new screen by giving it the screen name
Before moving on check out MIT’s website to see all the things you can do with text: Text blocks
Booleans
A boolean can be one of two values: true or false. True basically means “yes” and false basically means “no”. To get a boolean, go to logic in the built in functions and grab one of these blocks:
For now, there is not much to do with booleans other than keep them in mind. Booleans and logic will be covered in more detail in Code 3: Logic, Loops and Conditionals.
Variables
One of the most important concepts in coding is a variable. A variable is a name for a piece of data that can change in value. An easy way to remember this is that a variable can vary, or change in value. There’s lots of information that is important to you every day that changes value. Here are some examples:
- Your age
- Your height
- Your location
- Today’s date
To better understand variables and how to use them in App Inventor, let’s walk through an example. Imagine you are creating a game where the user can earn and lose points. You want to have a score for the player to see in a text box. At the start of the game, the player has zero points so you added a block like this to the game.
The player sees zero in the textbox that contains her score. However, the player’s score will change as she plays the game. Each time she gains or loses points you have to update the text box. When she gets more points, you add a block like this.
That works, but now what about the next time the player earns 10 points? You’ll have to update the text again to say 20. Then what if the player loses points? You will have to change her score to 15. What if she gains points again? This can get confusing really fast.
In scenarios like this, you can create a variable to keep track of the player’s score. You can create a variable called score that always has the value of the player’s current score. As she plays the game, you can add and subtract points from score and you won’t need to worry about keeping track of what the her current score actually is. Here’s how it would work in App Inventor. First you would grab this block from the variables section.
This block tells the app that there will be a variable and asks you to give the variable a name and a value. Here’s what the block would look like for a game where the player's score started at zero.
With this block, you are telling the app that there will be a piece of data called score and that it will change value as the game is played. Every time the app starts up, it is going to create a variable called score and set it zero. Now you can do what’s called “set” and “get” your score variable. “Get” will allow you to see what the value of the score is. Here’s how you would tell your app to display the score in a textbox.
“Set” allows you assign a new value for your user’s score. Every time the player earns points you can tell the app to do this.
This tells your app to change the value of the score to whatever it is now and add ten to it. If the current score is zero, the new score would be 10. If the current score is 25, the new score would be 35. Now you don’t have to worry about reassigning the score every time your player gains or loses points. The app will just take the current score and add 10 to it.
It is okay if you are still confused about variables! They are really hard to understand and the best way to understand them is by using them. You will use them in the coding challenge at the end of this unit and again over the next few coding units.
Thought Exercise
Pick one of your favorite apps and identify at least three spots where you think the app creators used variables.
Local and Global Variables
You may have noticed in the section above that the variable block said “global”. There are two types of variables, local variables and global variables. When you create variables, you’ll have to think about whether you want to use the variable at multiple places in your code, such as the score variable from above, or if you want to use it in just one place.
Global variables can be used anywhere. Like you saw above, the score variable needed to be used multiple times. You had to use it when the player earned points, when the player lost points, and to display it in the textbox. It could also be used for a high-score table. You can never reuse a name when using a global variable. If you did, your app won’t know which variable to use. Luckily App Inventor won’t allow you to create two variables with the same name. If you’re in doubt of which variable type to use, use global.
Local variables can only be used at one spot in your code. If you need a variable to keep track of something in just one place, use a local variable. The local variable will only be able to be used inside the “local variable” block and App Inventor won’t let you use it outside of it. Unlike global variables, you can reuse the names of local variables.
In the coding challenge, you’ll see examples of when to use global variables and when to use local variables. To learn more about global and local variables visit MIT’s website: Global and Local Variables.
Lists
There’s so much information out there in the world, it can get really confusing! Luckily we have ways of organizing data so that we can easily find it and use it. Can you think of any examples of how you organize information and objects in your life? Here are some examples of things you might do to organize things:
- Put a friend’s phone numbers in a contact list
- Put pencils in a pencil box
- Put clothes in a closet
Programmers uses lists to organize data in their programs. Lists can hold multiple pieces of data and they’re easy to get data from. You may have made a to-do list or a grocery list before, and lists in programming are very similar. In App Inventor, you can go to blocks and select “lists”. List look like this:
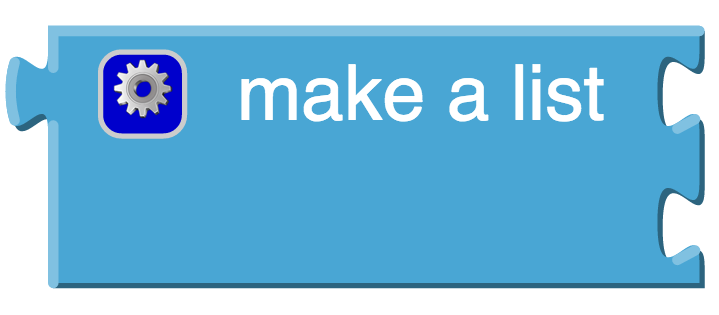
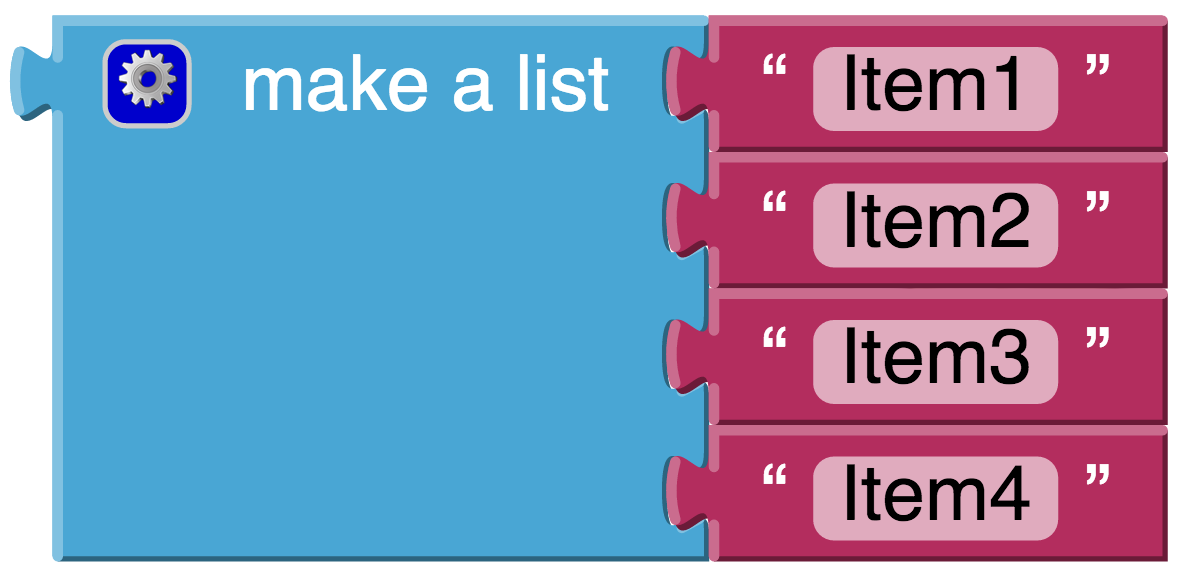
Lists are really useful for holding a lot of information. Each thing in a list has an index, which is a number that tells you where it is in the list. The first item in a list will have an index of 1, the second item in a list will have an index of 2 and so on. Here's an example:

Here is how your app sees this information:
List Name: Fruits
- Apples (Index = 1)
- Bananas (Index = 2)
- Oranges (Index = 3)
You can get one thing from a list instead of using the whole list. If you wanted to just put the string “bananas” into a textbox instead of the entire list, you would need to tell your app to look at index 2 in this list. Here’s how you would put “bananas” into a textbox using App Inventor, from the list shown above:
You can also add, remove, and replace things in lists. Let’s say you forgot to add “kiwis” and “grapes” to your fruit list. You could add them like this:
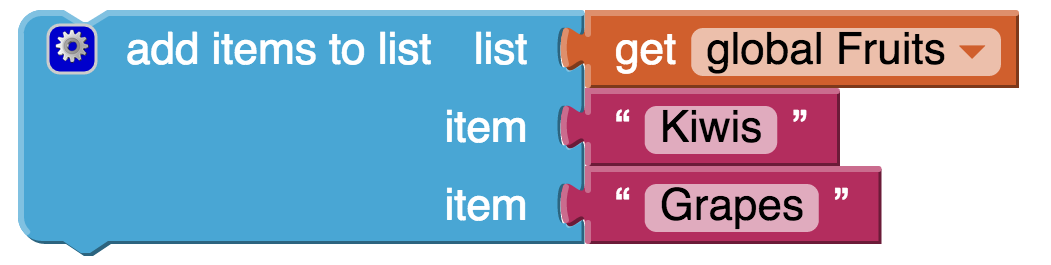
Now your app will see your list like this:
List Name: Fruits
- Apples (Index = 1)
- Bananas (Index = 2)
- Oranges (Index = 3)
- Kiwis (Index = 4)
- Grapes (Index = 5)
You should use lists anytime you have multiple pieces of information that you want to include under the same variable name. For example, if you wanted to display the top ten high scores for a game, you could make ten variables with names like HighScore1, HighScore2, HighScore3 and so on until you get to HighScore10.
The easier way to do this would be to make a list called HighScores that contains the ten high scores and then display them by using their indexes. Using a list would also allow you to replace and add more high scores more easily and would save you a lot of time!
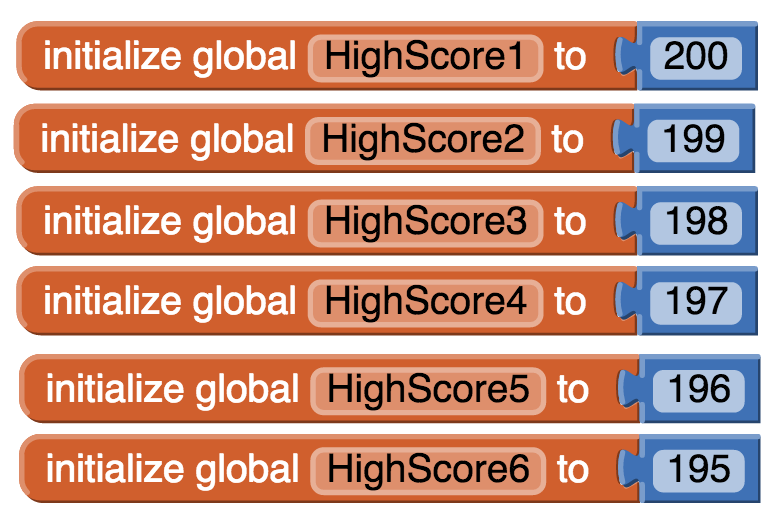
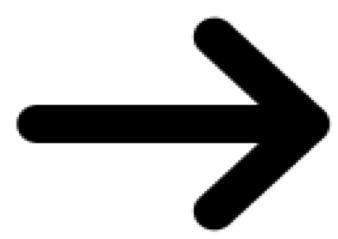
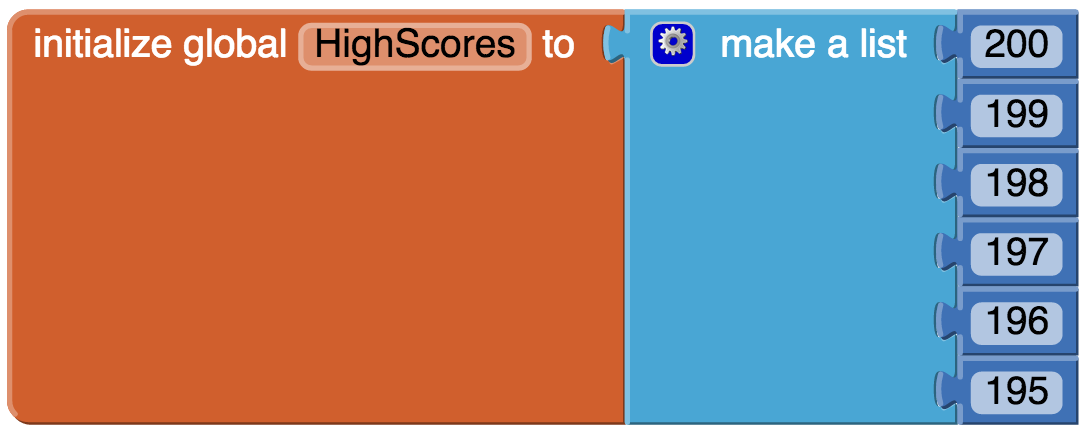
Check out MIT’s website for more information on lists.
Note: In many programming languages, lists are called arrays. and indexes start at 0, not at 1. Make sure to check the rules when learning a new programming language!
Databases
Variables are great for storing information while your user is using your app, but what if you wanted to store information between the times when your user opens and closes her app? You can do this with a database. Databases can store information for later use, even when the app is closed! You can think of databases as a place to store information you can recall later. Here are some examples of databases you may use every day:
- Contact list - you store your friend’s phone numbers so you can use them later
- Messaging app - most messaging apps automatically store old messages so you can reread them later
- Notebook - you write things down and read them later
In App Inventor, the component TinyDB allows you to save data on your user’s phone that will be there each time she logs into your app. It is important to know that TinyDB only allows you to store data locally. This means that two users cannot share data with the same TinyDB. When a user stores data into TinyDB, it will only be available on her phone, and no one else's. You will learn how to share information between phones in Code 4: Using Mobile Features & Connecting to the Web.
To use TinyDB, drag it onto your screen in the designer side of App Inventor. You can find it under the storage menu. It will appear as a non visible component and will look like this:
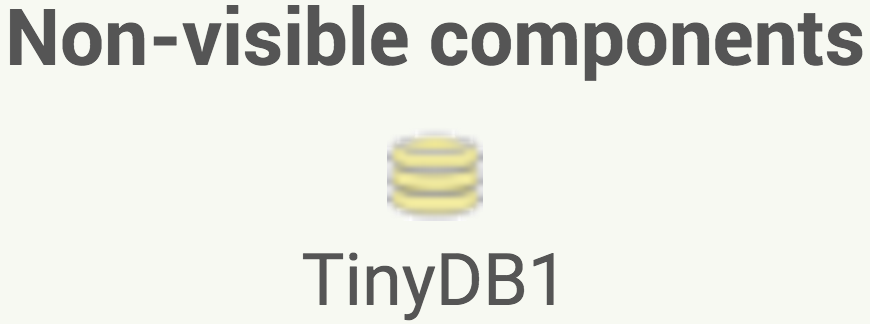
You can talk to your TinyDB by making calls to it. When you make a call, you can store things and get things from it. The way you store data in TinyDB is by giving it a tag. The tag is a name that you will use to retrieve the data. It is a lot like a variable name. If you use the same tag name to store data twice, the database will overwrite, or erase, the old data with the new data. This can be useful if you need to update what’s in your database but you should be careful never to repeat tag names otherwise! Here’s a few examples of how to make calls to TinyDB:
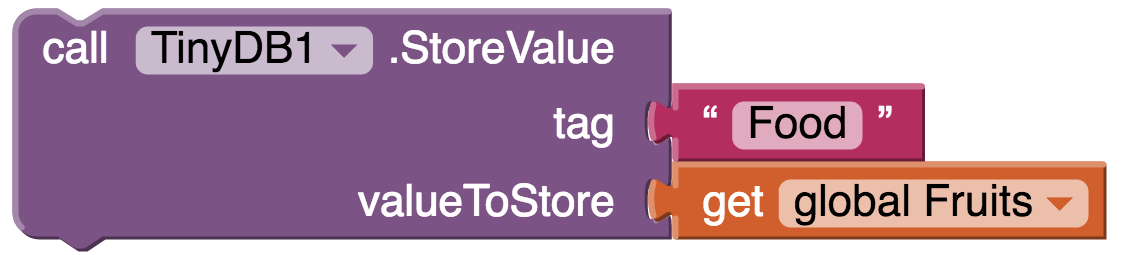
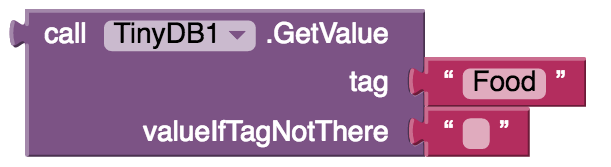
The first block shows how to store values in TinyDB. In this case we stored our fruits list from earlier with the tag “Food”. The second block shows how to retrieve the fruits list from the database. The tag needs to be typed exactly as it was when the data was stored, including all capital letters.
To better understand this, let’s walk through an example. Let’s say you needed to store three things in a database for later use. One is our Fruits lists from the last section, one of them is your age, and the other is a list of your favorite things to do. You make three to calls to the database like this:
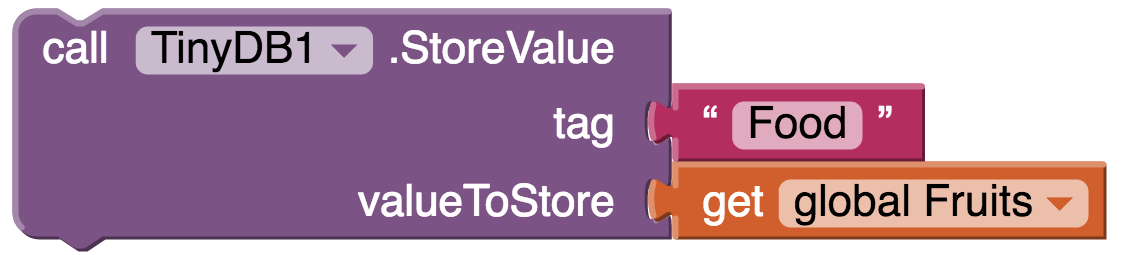
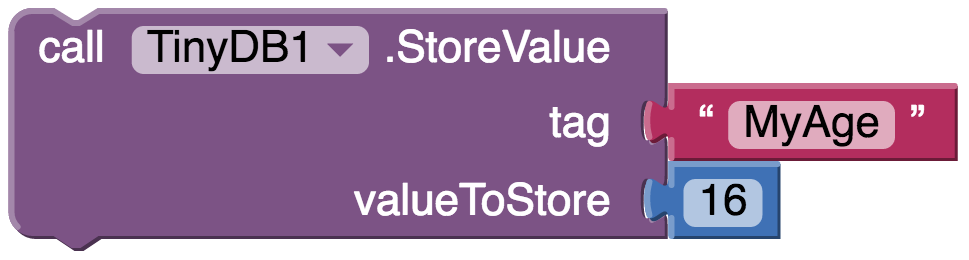
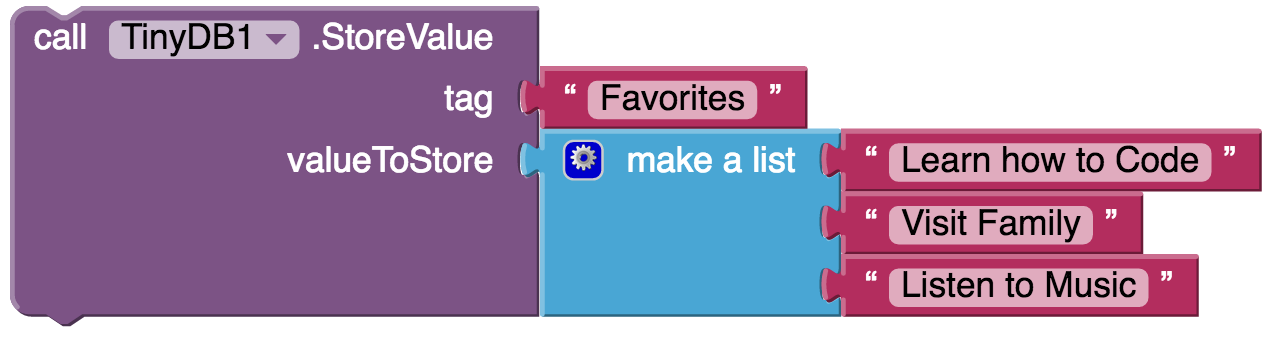
You now have three entries in you database, and this what they look like:
Tag Name: “Food”
Data:
- “Apples” (index = 1)
- “Bananas” (index =2)
- “Oranges” (index = 3)
Tag Name: “My Age”
Data:
- 16
Tag Name: “Favorite”
Data:
- “Learn how to Code” (index = 1)
- “Visit Family” (index = 2)
- “Listen to Music” (index =3)
Now, when you want to retrieve your favorite things to do, so you make a call like this:
When you use this block you will get “Learn how to Code Visit Family Listen to Music”. Now let's say you want to retrieve your age, so you make a call like this:
When you use this block you just get a blank string: “ ”. This is because the tag “myage” does not exist in your database! However, the tag “MyAge” does exists. Since your database did not recognize the tag, it defaulted to show you the block next to "valueIfTagNotThere", which is a blank string. You can make this string say anything you want. You can even make it be an error message to your user.
If you create an app with multiple screens (like your slideshow app), components and variables will not be able to talk to each other between each screen. You will need to use a TinyDB in order to transfer information from one screen of your app to another. Visit this page for more information: MIT Screens.
Congratulations on completing all of the content in this unit! It was a lot of information, so it’s okay if you don’t understand everything right now. You can always refer back to this unit when you are building your app. The Code Challenge is meant to incorporate everything you’ve learned so far, so give it a try!
Code 2 Challenge: Make a To-Do List
Description: Make a to-do list that allows users to enter in and remove items. Your app should remember the items each time your user opens the app. You may want to learn how to use a ListView before trying the challenge!
Try your hardest to do this challenge on your own before you read the instructions. Remember, these instructions are just one way the challenge can be solved! You can also download our example app in the Google Play Store. Happy coding!
Reflect
In this lesson, we covered a lot! You learned about all different data types and different ways to store and organize them. Here are some reflection questions for you to discuss with your mentor:
Q1: How does the information you learned in this lesson change the way you see apps you use every day?
Q2: What are some things from this lesson that you think will be useful in creating your app?
In this section you learned the following keywords:
- Data - Information that your app uses
- Data Type - The way data is categorized
- Number - A data type that is a number
- String - A data type that is text
- Boolean - A data type that is true or false
- Variable - A name for a piece of data that can change
- Global Variable - A variable that can be used at any point in your code
- Local Variable - A variable that is only used in a certain section of code
- List - A way of organizing multiple pieces of data
- List Index - A number that represents where data is in a list
- Database - A place to store and organize information
Additional Resources
Here are some additional resources on databases. You can use these now or if you need help when building your app!