MIT App Inventorでコードをデバッグし、エラーを修正するためのヒントを学ぶ
このレッスンのアクティビティ:
デバッグとは何か?
デバッグとは、コードが動作しない原因を突き止め、動作するように修正する作業のことです。
デバッグという言葉は、コンピューティングの先駆者であるグレース・ホッパー提督に由来しています。彼女は1940年代にハーバード大学でMark IIコンピュータに取り組んでいた、その際に蛾がコンピュータの中に引っかかってしまい、動かなくなってしまいました。
蛾を追い払い、彼女はシステムを「デバッグ」していると言い、その後プログラマー達はこの言葉を「自分のコードのエラーを修正する」という意味で使っています。

コーディングのヒント
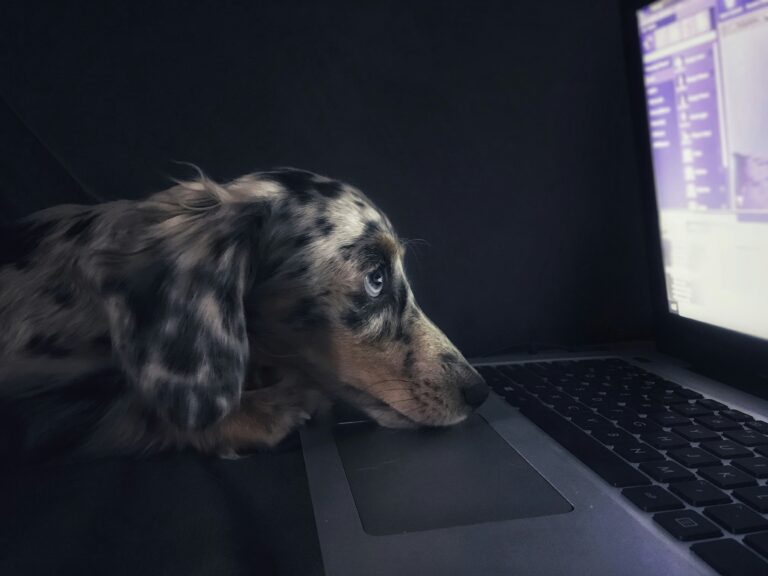
デバッグはコーディングの中で最も人をイラつかせ、時間のかかる作業であることが多いため、デバッグに十分な時間を割けるように計画することがとても大切です!
そして忍耐強く臨みましょう!
一歩一歩、少しずつ
プログラマーは時々、アプリ全体を一度にコーディングし、それをテストしたくなることがあります。
しかし、その誘惑に負けてはいけません!!!
何かが正しく機能しないことはよく起き、エラーの修正は何から手をつければいいのかを見つけることは困難なことが多くあります。
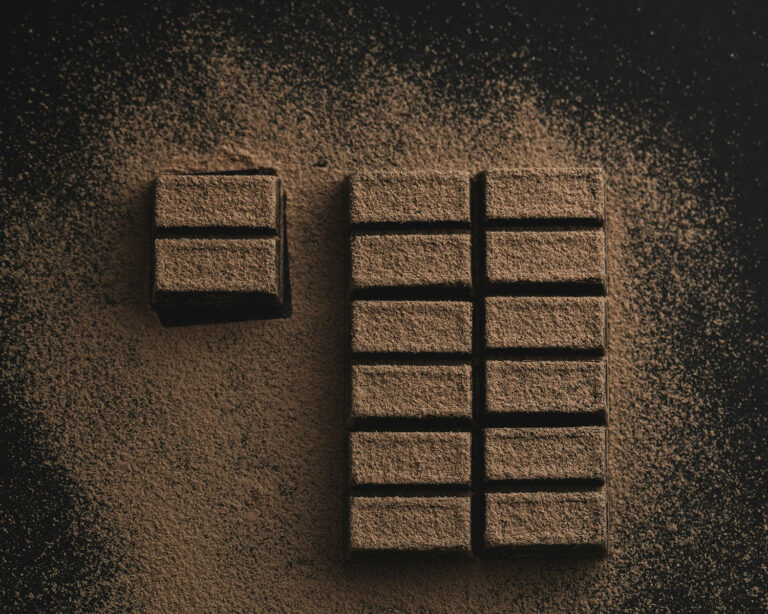
アプリのコーディングを小さな塊に分割しよう。
次のことに取りかかる前に、ひとつのことをやり遂げよう。
少しコードを書き、少しテストし、うまくいくことを確認したら、また少しコードを書き、また少しテストする、それを繰り返そう。
例えば、あなたのアプリに5つのボタンがあり、そのすべてが異なる機能を持っているとすると、
- 最初のボタンの動作のコードを書き、
- その後、動作するかどうかテストします。
- 2つ目のボタンに移り、
- 動作のテスト...と工程を繰り返します。
もしコードにエラーがあった場合、5カ所それぞれに戻って修正するよりも、1カ所づつ修正した方が簡単です。
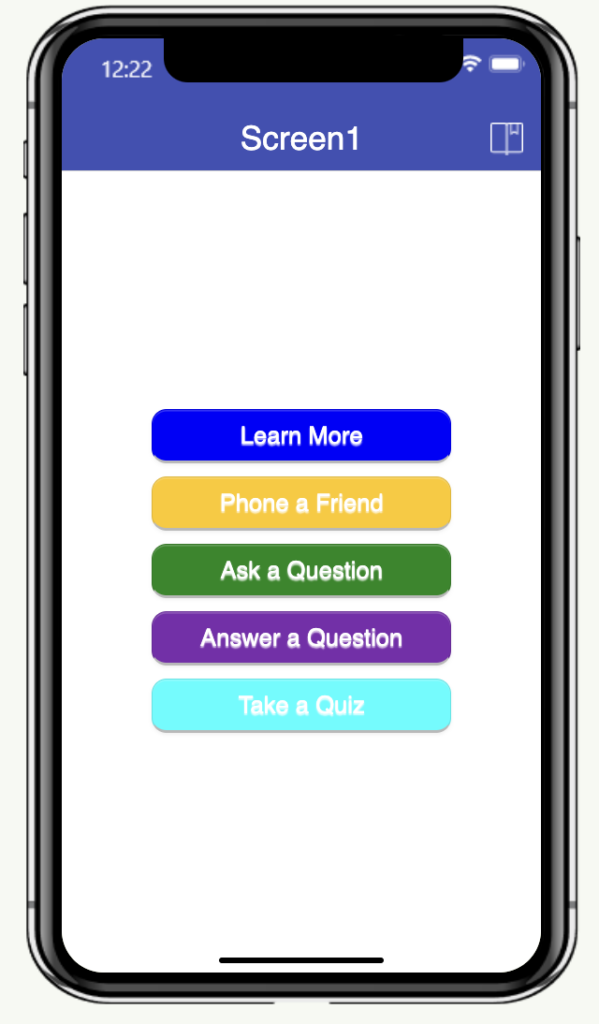
バージョン管理
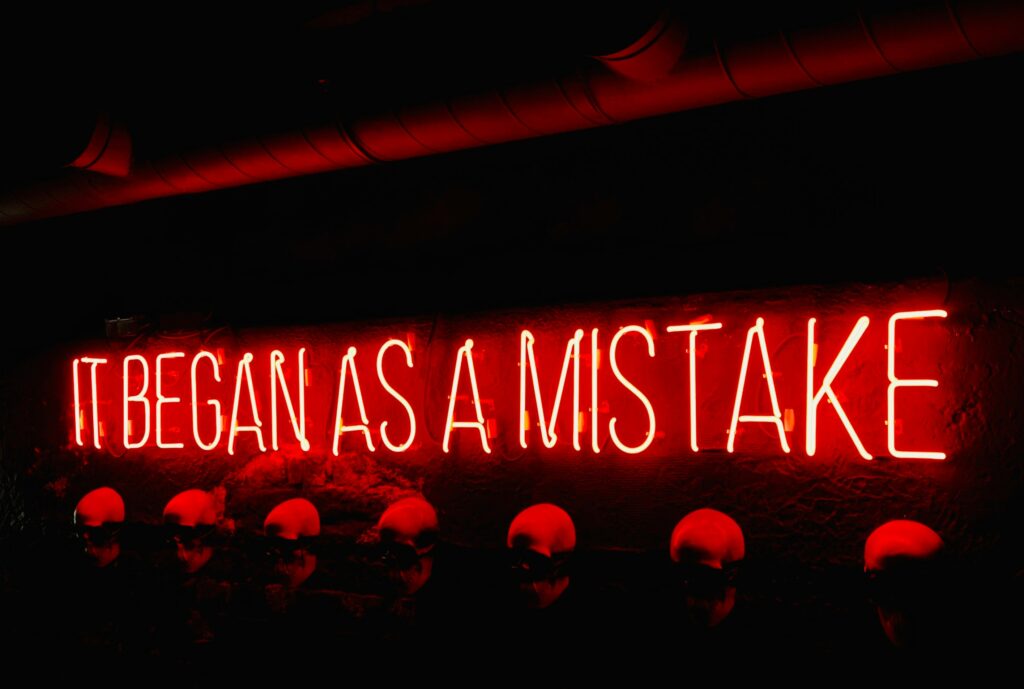
「小さなかたまり」ルールに従い、アプリの一部分を作り、動作させました。
しかし、新しいコードを追加すると、突然すべてが機能しなくなってしまいました。
オーノー!
デバッグのために、新しいコードを削除してみても、アプリはまだ動来ません。何がいけなかったのかわからず、「元に戻す」ボタンがあればいいのにと思うでしょう。
このようなシナリオを避けるには バージョンを保存することができます。
そうすれば、エラーが出てしまって、修正方法がわからなくなってしまっても、最後に保存したバージョンに戻ることができます。
また、バージョン管理を使えば、作業中のアプリを壊す心配をすることなく、新しい機能を試すこともできます。
App Inventorで異なるバージョンを保存するには、「名前を付けて保存」と「チェックポイント」の2つの方法があります。
その"チェックポイント"オプションは、あなたがつけた名前でコピーを保存しますが、あなたはオリジナルで作業を続けます。このアイデアは、動作するバージョンを「チェックポイント」し、プロジェクトのメインバージョンで開発を継続することです。
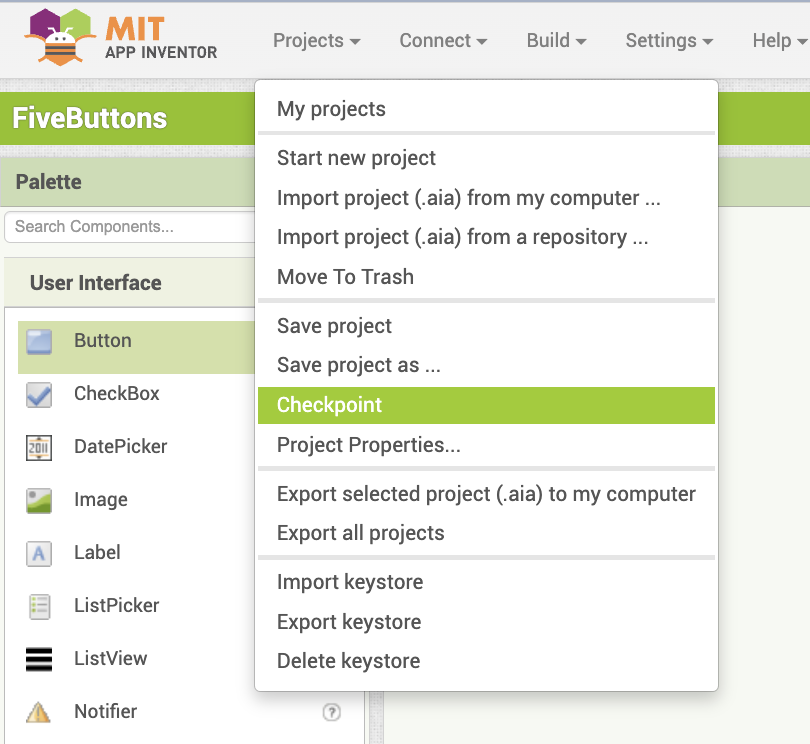
プロジェクトを 「プロジェクトを別名で保存 オプションは、作業中のコードのコピーを作成し、新しい名前で保存します。編集した内容はすべて新しいコピーに反映されます。
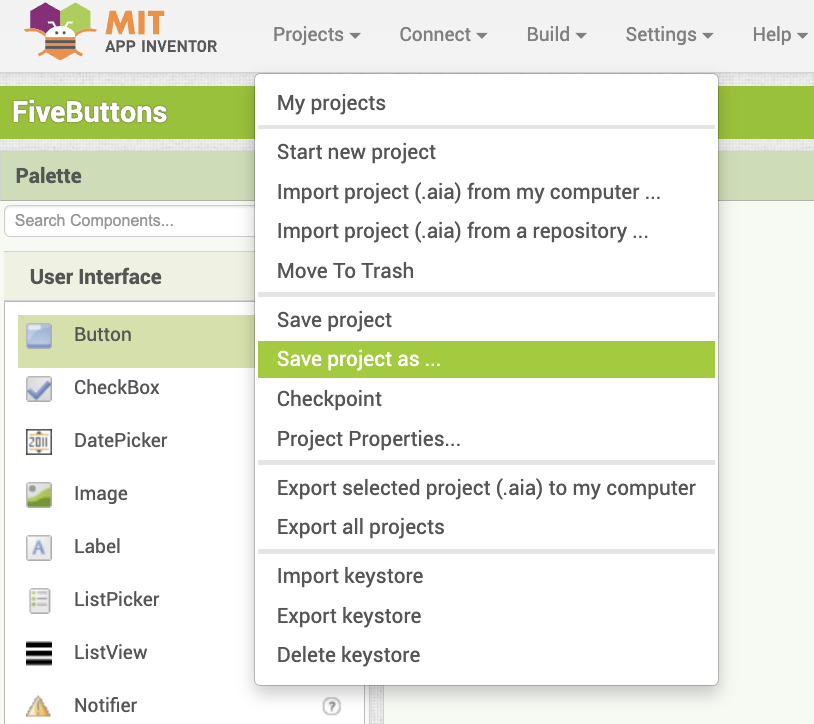
ブロックの折りたたみ
ブロックの折りたたみは、コードのワークスペースがブロックで混雑し始めたときに、コードを整理するための方法です。
ブロックを右クリックすると、ブロックを折りたたむオプションが表示されます。
ブロックを再び展開するには、ブロックを右クリックして「Expand Block」を選択します。
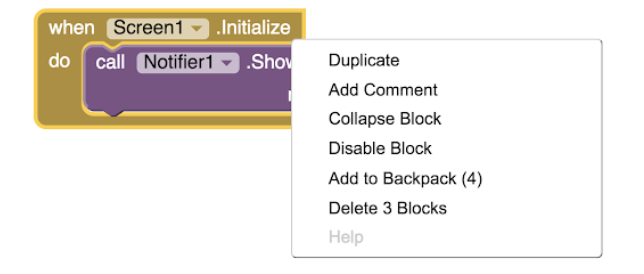
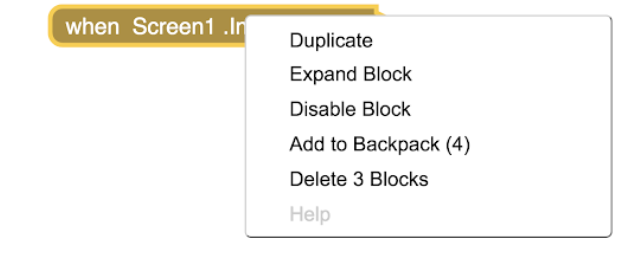
ブロックを折りたたむと、画面上のバーが1本に圧縮されます。
折りたたんだブロックは、スペースを取らなくなったでけで、通常のブロックと同じように使うことができます。これは、編集する必要がなくなったブロックを折りたたんで、画面をすっきりさせたい場合に役立ちます。

ブロックの無効化
ブロックを無効にすることもできます。
画面には残りますが、アプリ内では実行されません。
ブロックの無効化を使うことで、特定のコード・ブロックなしでアプリをテストすることができます。デバッグをしてエラーがなくなった後に有効にすれば、今まで通り実行することもできます。
また、Notifiersのように、テストにのみ使用しているブロックを無効にすることもできます。
App Inventor でブロックを無効にするには、次の 2 つの方法があります。
イベント・ハンドラ・ブロックの外にブロックをドラッグするだけで、それらのブロックを無効にし、実行できないようにすることができます。それらはイベントの一部ではないので、実行されることはない。いつでもスナップインして使用することができます。
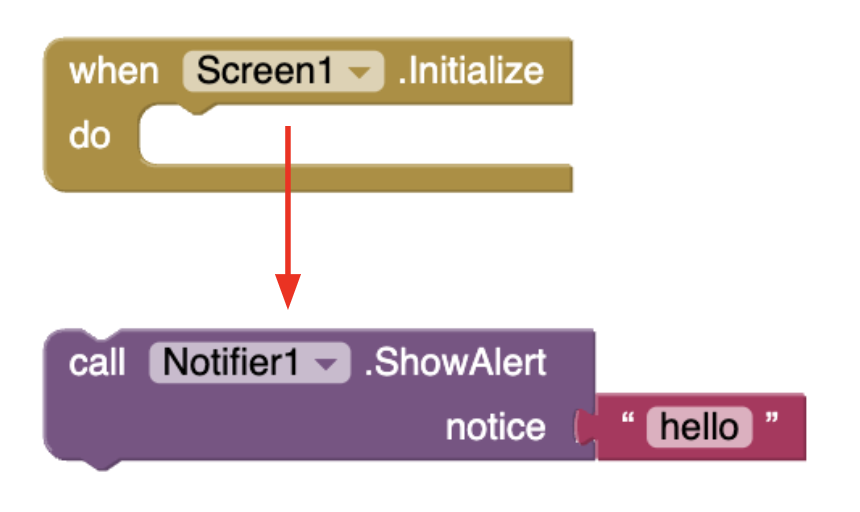
ブロックまたはブロックのグループを右クリックして、「ブロックを無効にする」を選択することもできます。ブロックは灰色で表示され、実行されないことを示します。このようにして、ブロックのセット全体を無効にすることができます。
ブロックを再び有効にするには、右クリックして「ブロックを有効にする」を選択するだけです。

コメント
プログラマーはしばしば、コードにコメントを残して、それが何をするのかを説明します。
コメントは、チームメイトやメンター、審査員など、他の人があなたのコードを見るときに役立ちます。
また、後でコードに戻ってきたときに、コードのどの部分が何をしているのか忘れてしまった場合にもとても役立ちます。
コメントを追加するには、ブロックを右クリックして「Add Comment」を選択します。
その後、ブロックの隅に?マークが表示され、テキストを追加することができます。また、?マークをクリックすると以前書いたコメントを見ることができます。
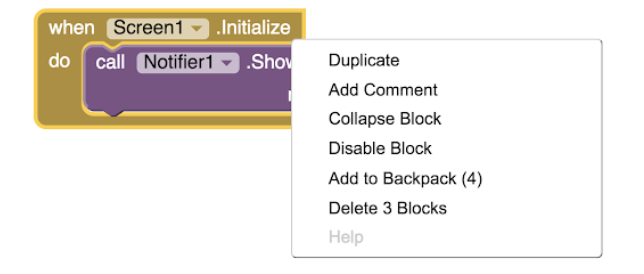
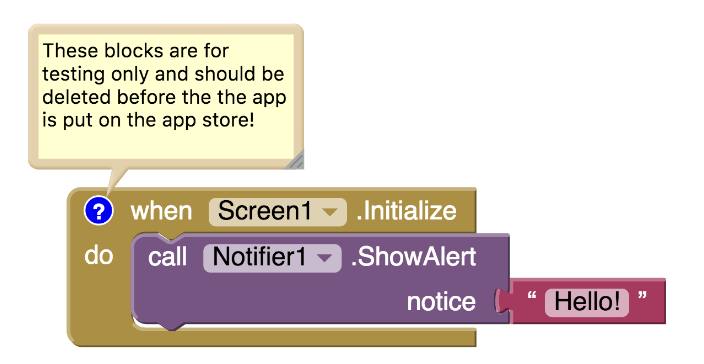
Tip
ベストプラクティスプラクティス コメントはプログラミングにおいて最も役立つものだ。
コーダーが何かをコーディングした後、眠りにつき、翌朝戻ってくると、前の晩に何をしていたのかまったくわからないということが何度もあった。必ずコメントを書くこと!
It’s also a great way to check your knowledge, because if your coding blocks are easy to comment on then you must understand them really well! Another thing to be aware of is that <, >, =, ≤, ≥ are hard for students for the first time (and for programmers who are years into their careers). When we set a math statement to < when it should be ≤ this is called an “off by one” error and this is a problem that happens to developers with 20 years of experience 😛 It is a very popular problem so be on the lookout for that.
デバッグの最良のタイプは、ラバーダック・デバッグだ!これは、あなたが本当にコードに行き詰まっていて、それが意味をなさない場合です。誰かに問題を指摘してもらえるかどうか、時間をかけて話し合いたいところだが、あいにく周囲には誰もいない!そこで、あなたは信頼できるゴム製のアヒルをデスクに置き、アヒルの友達に声を出して問題を説明する。彼らが理解できるように、とても簡単な言葉で説明しなければならない!私の同僚は皆、デスクにラバーアヒルを置いて、よくこれをやっている!
生徒への質問経験豊富なコーダーがバグをデバッグするのにどれくらいの時間がかかると思いますか?しかし、各大手サイトには、バグがどれくらい存在するかを見るためのページがあり(例えばFacebook)、また、ページがダウンしているか、壊れているか、現在デバッグ中かどうかも表示されます(Facebookの例)。
どうやってバグを見つけるのでしょうか? (たくさん、たくさんテストすること!)どのくらい組んだコードをテストをするべきでしょうか?他の人にどのくらいのテストをやってもらうべきでしょうか?
バグを発見したとき、どうすればそれを知ることができますか?時々、画像をクリックし続けて、クリックするはずのボタンが画像の下にあることに気づくことがあります。それはバグでしょうか?アプリメーカーはバグだとは思っていませんが、あなたにとってはバグかもしれません。
メンターTipsは、AmeriCorpsから提供されたものです。
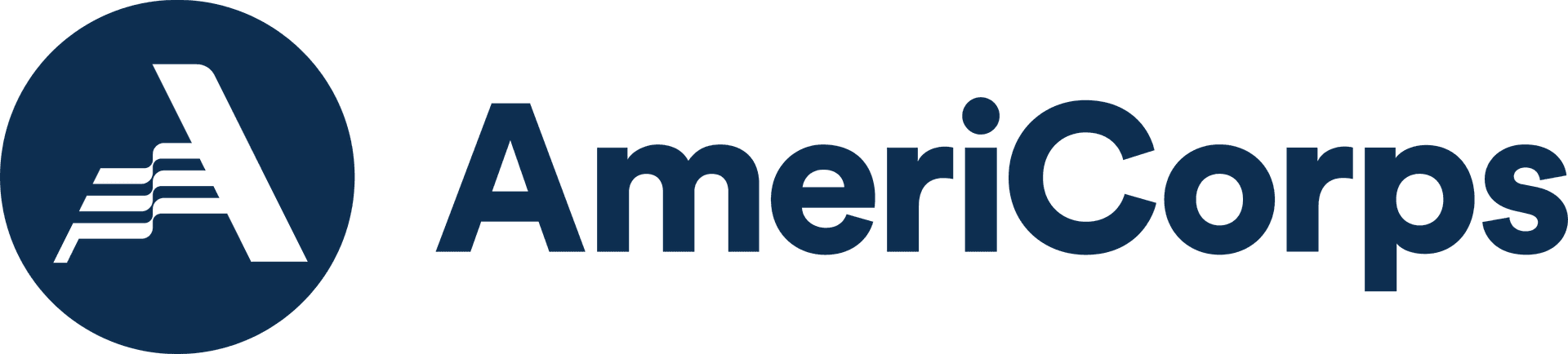
デバッグのヒント
ノーティファイアとラベル
通知 ノーティファイアコンポーネントは、コードが壊れた原因を突き止めるのに役立つ。
App Inventor アプリケーションに Notifier を配置するには、ユーザ インタフェース パレットからドラッグします。
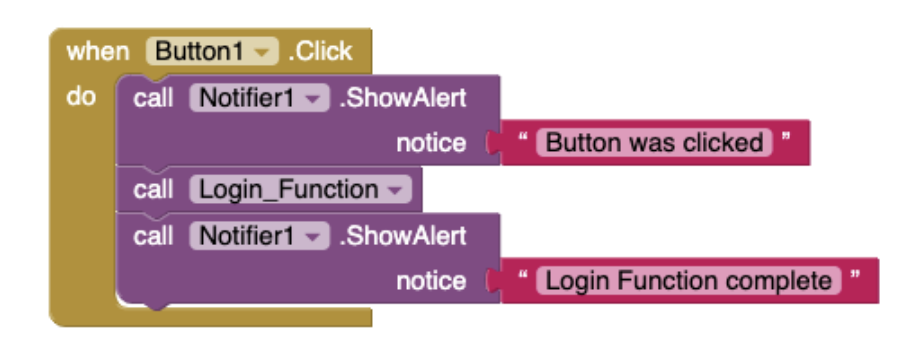
Notifierを使う最も簡単な方法は、特定のブロックが実行された(または実行されていない)ことを確認するための情報を持つShowAlertブロックを追加することです。
メッセージだけでなく、変数の値やImageSpriteの位置などの情報を表示することもできます。
を追加することもできます。 ラベルを追加することもできます。
例えば、アプリの実行中、特定のイベントが発生したときに、変数の値を知りたい場合があります。
Label.Textに知りたいことを設定すれば、アプリに表示することができます。

エラーをデバッグしたら、ラベルを不可視にするか、アプリから完全に削除しましょう。
実行する
そのする 機能もデバッグに最適なツールだ。
携帯電話とAIコンパニオンアプリ、またはエミュレーターを使用してアプリをライブテストしている間、新しいコマンド「Do It」にアクセスできます。
このコマンドは、Connectメニューから電話またはエミュレーターに接続するまでグレーアウトしています。
Do itを使えば、アプリ自体を操作することなくブロックを実行できるので、変数や要素の現在値を見ることができる。
グローバル変数の取得」ブロックをドラッグして右クリック。ドロップダウンメニューから「実行」を選択すると、そのブロックが実行される。
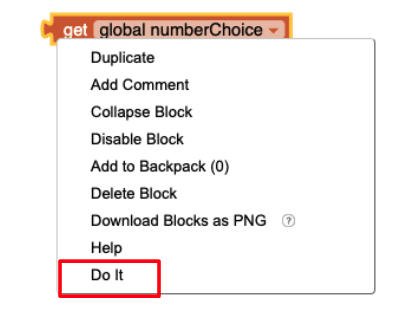
結果が表示されたボックスの上に黄色いDo Itボックスが表示されます。これはどのコンポーネント・プロパティにも使えます!
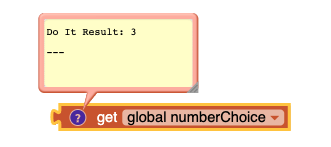
Do Itを使ってアプリ内の何かを変更することもできます。例えば、リスト内の項目を変更したいとします。そのためのブロックをドラッグし、Do Itを選択します。結果は表示されませんが、リストはアプリ内で更新されます。
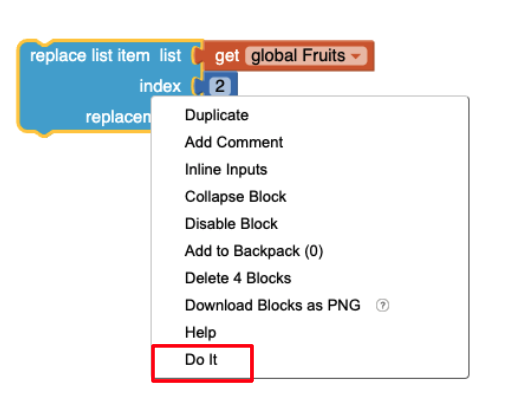
アプリ内の既存のコードブロックを実行することもできる。
例えば、ここではDo Itを使ってListViewの要素を更新する。それがアプリに反映される。ListViewには、Do Itコマンドで設定された新しい要素が表示されます。
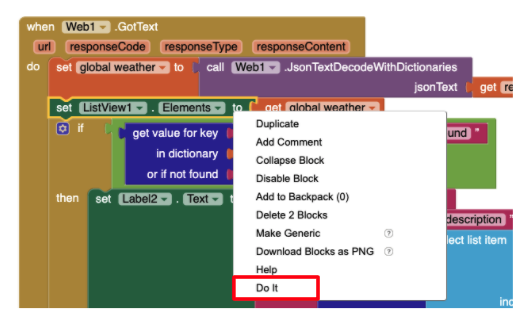
テストデータを使用する
アプリで使用する情報が多い場合は、テストデータを使用すると便利です。テストデータは、アプリが正しく動作していることを確認するために使用できる、よりシンプルで小さなデータセットです。
例えば、近くのレストランを表示するアプリを作っている時、レストラン情報を保存するためにGoogle Sheetsを使っているとします。

1つか2つのテスト用のレストランから始めて、アプリが機能するかどうかテストすることができます。
テストが完了したら、レストランのデータ一式をGoogleシートに追加します。
プロパティを変更する
アプリをライブテストしている場合、アプリを実行しながらDesignerでプロパティ値を変更し、その効果を確認することができます。
これで問題を明らかにすることもできます。
例えば、あなたがゲームをコーディングしている時のことです。スプライトが動作している際、スマホではスプライトの位置が正しく見えないことがあります。
ライブテスト中は、以下のことが可能です。
- Designer画面に移動します
- スプライトのXとYの値を変更します
- 実行中のアプリの画面上のスプライトの位置が変わります
これを使用して、画面上の座標をテストし、理解することができます。

アクティビティ1:バグを修正する
タイマーアプリのバグ修正
- App Inventorにアプリをロードします。
- アプリをテストするためにエミュレータまたはデバイスに接続します。
- Try pressing Start without typing anything into the textbox. What happens?
- 秒数に0または負の数を入力してみてください。どうなりますか?
- 条件ブロックを使用してバグを修正しましょう!
アクティビティ 2:コメントを追加する
App Inventorプロジェクトにコメントを追加する
ブロックのグループが何をするのかを説明するコメントを、少なくとも1つアプリに追加してください。あなたが最も理解しにくいと思うブロックグループを選んでください。なぜなら、そのブロックはおそらくチームメンバーなど他の人にとって最も理解しにくいブロックだからです。
振り返り
これらのヒントは、Technovationプロジェクトでモバイルアプリのコーディングを始める際に役立ちます。
だが、覚えておいてほしい。

主な用語のおさらい
- デバッグ - コードが動作しない原因を突き止め、それを修正するためにコーダーが行うプロセス。
- バージョン管理 - 進捗に応じてアプリの作業バージョンを保存
- テストデータ- アプリが正しく動作していることを確認するために使用できる、よりシンプルなデータセットです。
- コメント - 何をするのかを説明するためにコードに含まれるテキストです。
追加リソース
App Inventorのデバッグ・リソースをいくつかご紹介します。