- Learn about event-driven programming
- Understand the different types of blocks in the Thunkable platform
These are the activities for this lesson:
EVENTS
Mobile apps are based on event-driven programming.
Event driven programming is based on events, rather than running code from top to bottom. Some programs just execute all their code at once, but mobile apps work based on how the user interacts with it.
Mobile apps work based on events, which are things that happen to trigger code to run. An example would be – the user clicks on a button. The event is when the user clicks. And the code to run when that event happens is called the event handler.
STOP AND DISCUSS
Can you think of some ways you interact with your phone and what your phone does? Here are a few to get started:
- When you click an icon for an app, the app opens.
- When you click “send” for a text message, it sends the message and makes a sound.
- When you try to log into an app, the app asks for a password.
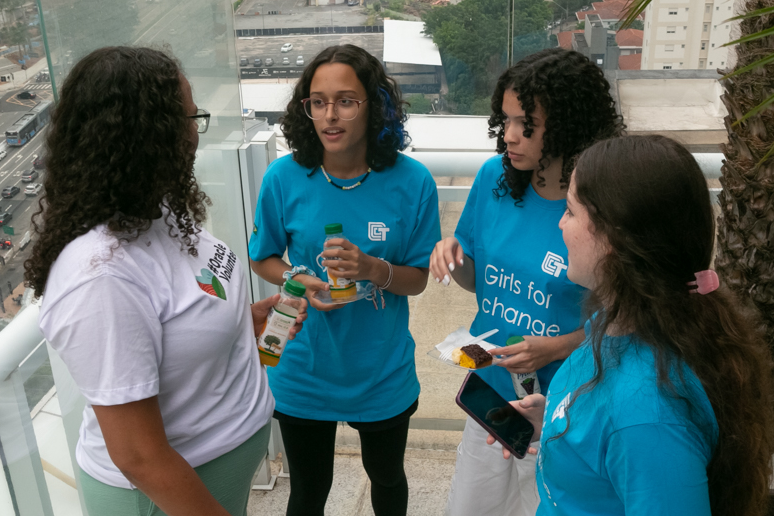
Let’s start by looking at the different parts of the Thunkable platform.
Thunkable has two windows you use to build your app. Clicking on Design in the top menu bar (top left of screen) takes you to the Designer, where you design the user interface. The user interface is everything in your app that a user can interact with. These things can be buttons, navigation bars, text boxes, pictures, etc.
THUNKABLE DESIGNER WINDOW
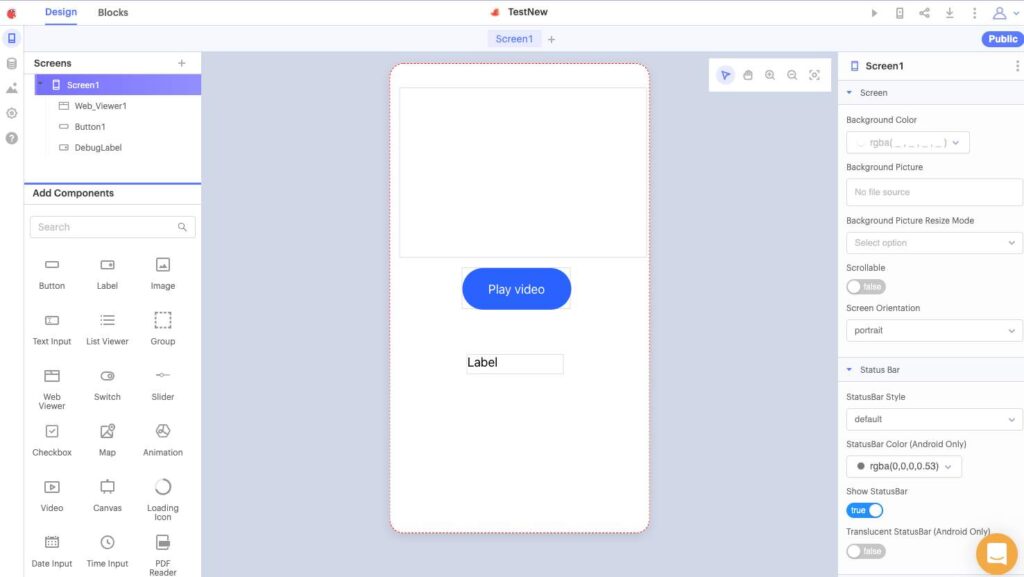
Component List
All components you have added to your app appear here, organized hierarchically by screen.
Workspace
You drag your components onto the phone in this central workspace. You have control over where the components are placed as well as their size.
Add Components
You drag visual components from this panel into the workspace.
Properties Panel
The Properties panel allows you to set the properties for each component. Properties are different characteristics you can set for each component, like its width, height, and color. Select a component on the mockup and then you can change any of its properties in the Properties panel.
When someone uses your app, they will interact with your user interface, by clicking buttons, entering text etc. It is up to you to decide what your app should do and to program it to do those things.
BLOCKS EDITOR
Clicking on “Blocks” takes you to the Blocks Editor window, where you do all your coding. You drag blocks from the panel on the left into the workspace in the center of the screen.
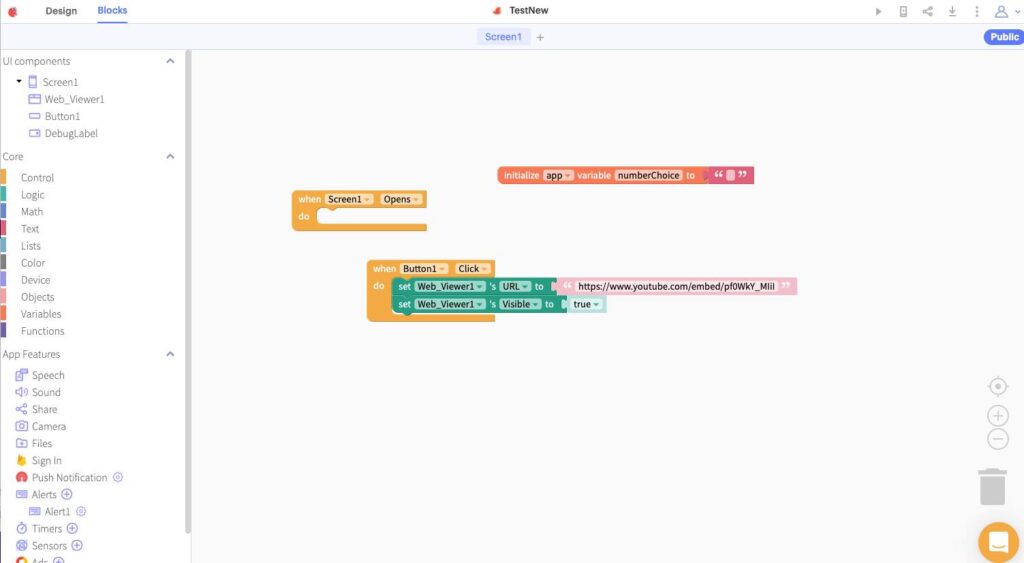
On the left, you will find the blocks palette. At the top are the UI components. Each component in your app has its own set of blocks. You will click on the component to show the blocks you can drag into the workspace.
Coding Workspace
This is your workspace where you drag all your blocks. You can move them around, snap them to and into each other. You can also delete any blocks you don’t need.
Core Blocks
The core blocks are standard coding blocks to use in your app. They are categorized according to their type and color coded.
App Features
These are invisible features you can add to your app. You can click on one to add it, and then set properties and drag out code blocks for that feature.
EVENT HANDLERS
Event handler blocks in Thunkable are a gold color and are shaped as an open block, so you can snap blocks inside of it. Those blocks run only when that event happens.
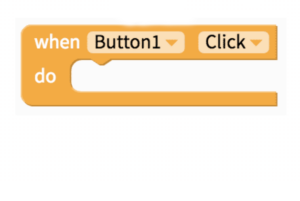
When Button is Clicked
You’ve already used this. When the user clicks a particular button, you would want the app to do something, like open another screen, or send a message.
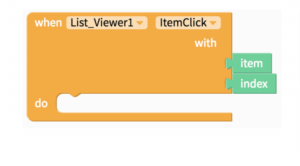
When ListViewer Item is Clicked
A ListViewer is like a dropdown menu, so this event happens when the user chooses an item in the list. The app should do something with the item that is clicked.
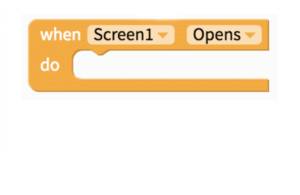
When a Screen opens
This event is used when you want to do something when the app first starts or the app switches to another screen. You can set variables, or update information from the cloud.
FUNCTIONS
Functions are blocks of code that do something. They can be run many times within an app. In Thunkable, functions blocks are colored purple. Some languages refer to functions as methods, or procedures. They do something, so you can think of them as action blocks.

Say
This function lets the app say or speak whatever text you want it to speak.
Timer Start & Sound Play
The Timer component can start counting. And the app can play a sound.
SETTERS AND GETTERS
Green blocks relate to the component’s properties.
The light green blocks are called getters, because you are getting the value of the property.
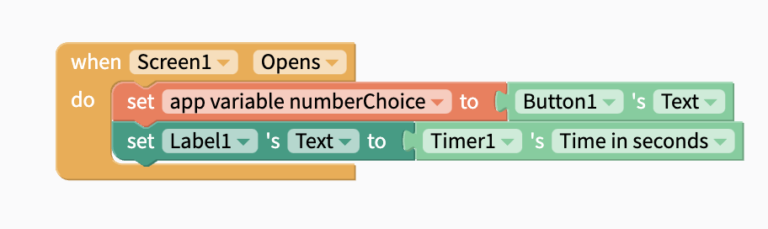
Text of Button1
The Text is gotten and saved in the variable numberChoice.
Timer1's Time in seconds
The value of the timer's seconds is gotten and stored in Lablel1's text, so the value is displayed in the label.
Setters are darker green, and they can be snapped to, with an open slot on the end. This allows you to set the value of the property.
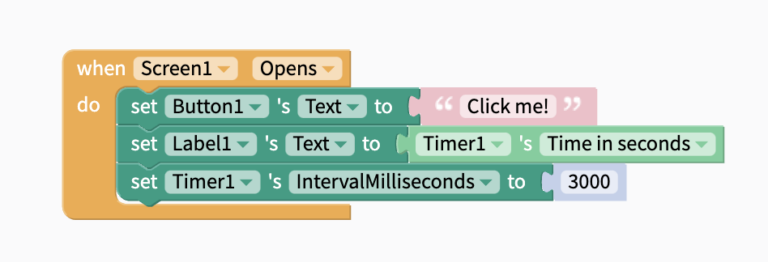
Text of Button1
Button1’s Text is set to the words “Click me!”
Label1.Text
Label1’s Text is set to Timer1’s Time in Seconds.
Timer1's IntervalMilliseconds
Timer1’s IntervalMilliseconds (how often it goes off) is set to 3000, or 3 seconds.
Mentor Tip
Best practices: Remind the students that this is exactly like what real coding is like. The weird vocabulary (functions, getters, setters) that we are using is exactly what coders use.
Guiding Questions to ask students: Can you think of some day-to-day functions you do? (example: baking a cake, brushing your teeth – anything that has repeatable steps). Functions are very similar to algorithms!
Mentor tips are provided by support from AmeriCorps.
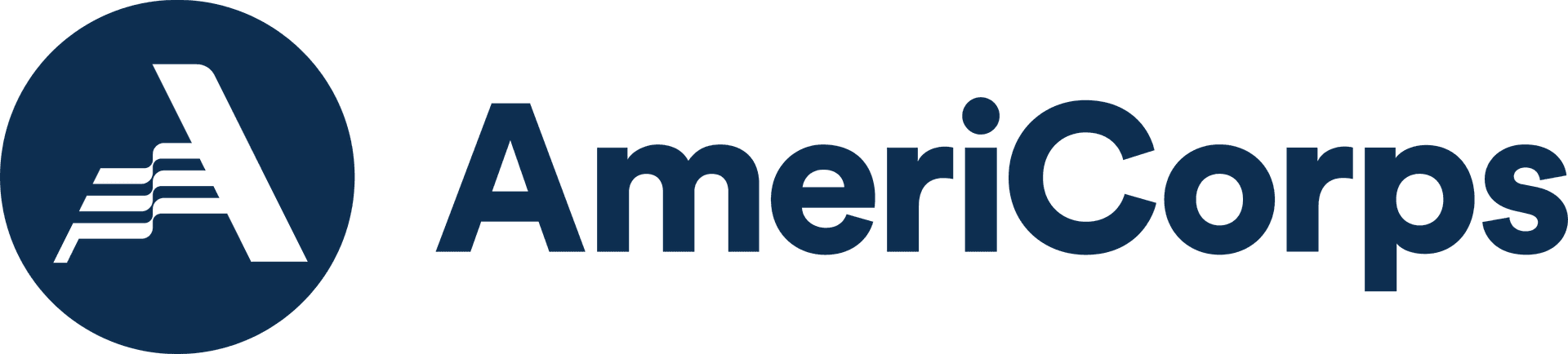
DATA BLOCKS
When you use setter blocks, you might use some other built-in blocks that represent data, or information that can be used in your app. Some examples of data blocks that can be used are seen below.
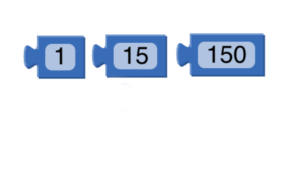
Numbers
Number values can be used as data in an app. These blocks can be found in the Math drawer in the core section of the blocks palette.
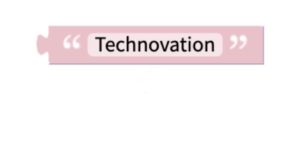
Text or Strings
Text, sometimes called strings, can be used as data. These are letters, words, and sentences that can be used in an app. The blocks are found in the Text drawer of the Core section of the blocks palette.
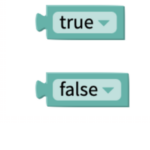
Boolean
This data type has only two possible values – true or false. These blocks can set or test the “state” of something and will be used in conditionals, which you will learn more about.
ACTIVITY: SOUNDBOARD TUTORIAL
Follow the video tutorial below
Then follow Dave's video below to use Event Handlers and Functions to play speeches in your app.
CHALLENGE
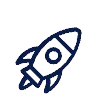
In the activity, you have used:
- Event Handler blocks
- Function blocks
Let’s try using some setter and getter blocks by adding to your Soundboard app.
When the user clicks on any picture to play the associated speech, change the background color of the screen.
You can change it to one particular color, but then you cannot easily change it back to the starting color, black, so instead, change the screen’s background to a random color.
Hint: Check the Color drawer to see how to get a random color.
REFLECTION
Now that you’ve learned a little bit more about the different code blocks, think about your app solution:
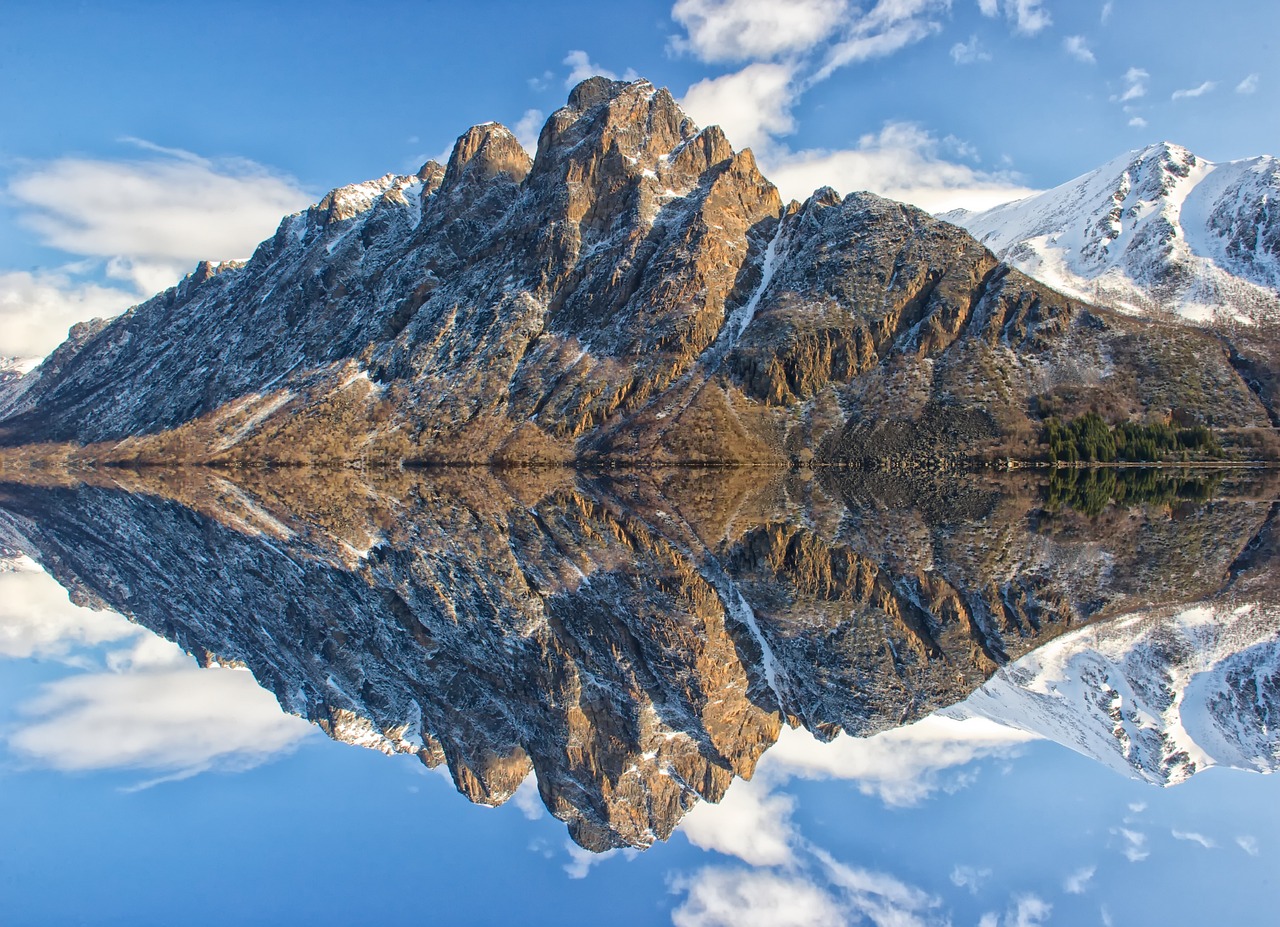
REVIEW OF KEY TERMS
- User Interface – everything in your app that a user can interact with
- Designer – window where you can add components to your app and design how they look
- Blocks Editor – window where you code the blocks for your app
- Event – something that happens to trigger code to run
- Event Handler– code blocks that tells your app what to do when an event happens
- Event Driven Programming – programming based on events, rather than running entire code from top to bottom
- Functions – a block of code that runs, and can be run multiple times
ADDITIONAL RESOURCES
Check out more of Dave Wolber’s Thunkable tutorials at draganddropcode.com
Student Ambassador Meenakshi Nair’s free Thunkable course on Udemy is another great resource!