- Learn about event-driven programming
- Understand the different types of blocks in the MIT App Inventor platform
These are the activities for this lesson:
EVENTS
Mobile apps are based on event-driven programming.
Event driven programming is based on events, rather than running code from top to bottom. Some programs just execute all their code at once, but mobile apps work based on how the user interacts with it.
Mobile apps work based on events, which are things that happen to trigger code to run. An example would be – the user clicks on a button. The event is when the user clicks. And the event triggers code to run, called the event handler.
STOP AND DISCUSS
Can you think of some ways you interact with your phone and what your phone does? Here are a few to get started:
- When you click an icon for an app, the app opens.
- When you click “send” for a text message, it sends the message and makes a sound.
- When you try to log into an app, the app asks for a password.
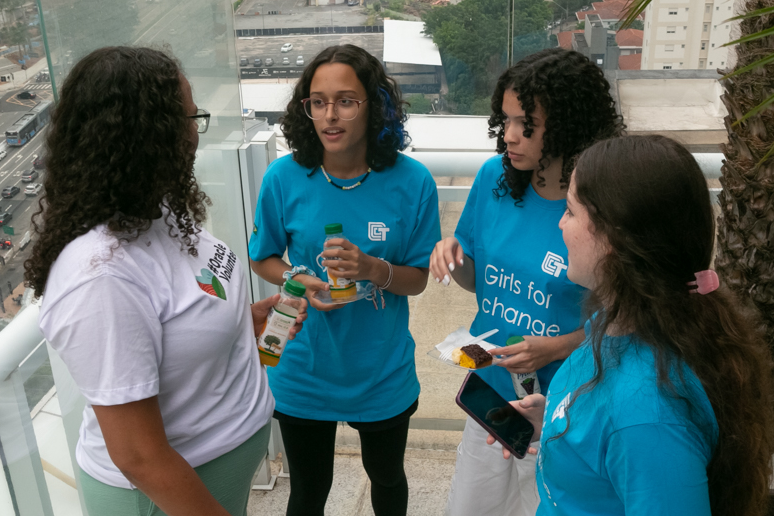
Let’s start by looking at the different parts of the App Inventor platform.
App Inventor has two windows you use to build your app. Clicking on the Designer button (top right of screen) takes you to the Designer, where you design the user interface. The user interface is everything in your app that a user can interact with. These things can be buttons, navigation bars, text boxes, pictures, etc.
APP INVENTOR DESIGNER WINDOW
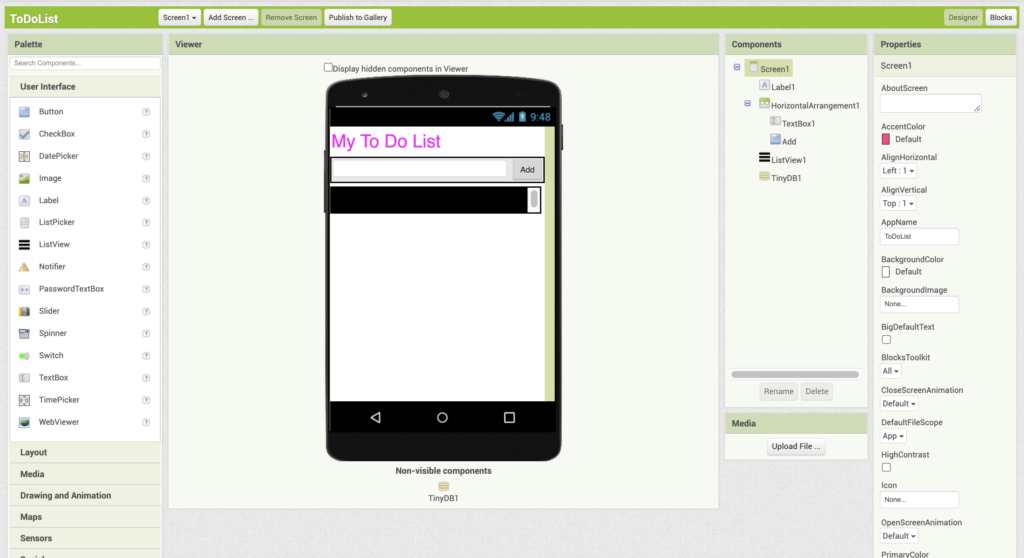
Palette
You choose components from the Palette to add to the Viewer, which is in the middle of the window. There are several drawers of components to explore and use.
Viewer
You drag your components onto the Viewer. Components are added from the top down, on the left. You have to use Layout components and alignment to get more control over exactly where your components appear.
Components List
This list gives you a heirarchical list of the elements on your screen. You can select them by clicking on them in the Viewer or in this list.
Properties Panel
The Properties panel allows you to set the properties for each component, like font size, alignment, color, etc.
Non-visible components
Non-visible components won’t appear on the screen but are still part of the app. They appear below the screen when you drag and drop them.
When someone uses your app, they will interact with your user interface, by clicking buttons, entering text etc. It is up to you to decide what your app should do and to program it to do those things.
BLOCKS EDITOR
Clicking on “Blocks” takes you to the Blocks Editor window, where you do all your coding. You drag blocks from the panel on the left into the workspace in the center of the screen.
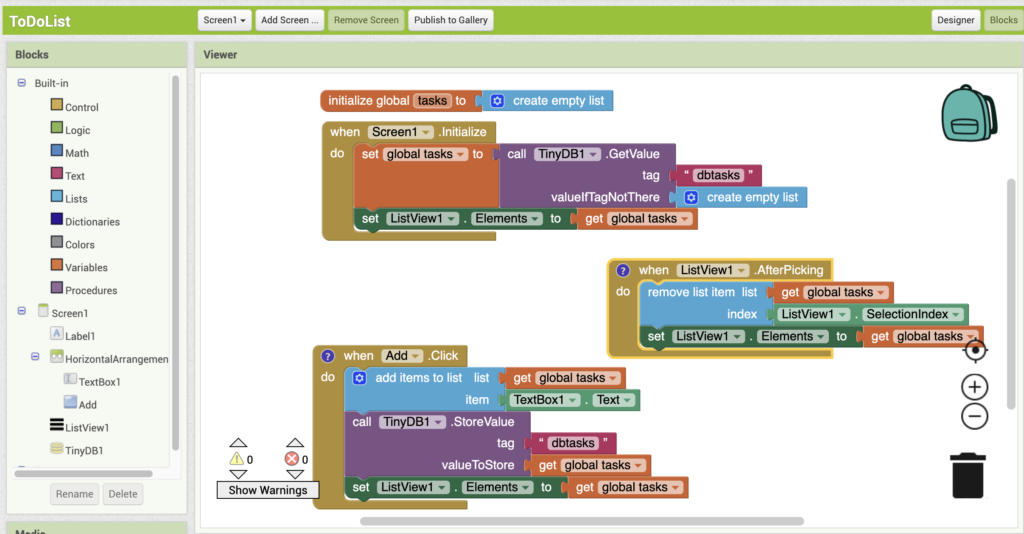
On the left, you will find the blocks you can drag into the workspace. They are in categories/drawers like Control and Logic.
Viewer
This is your workspace where you drag all your blocks. You can move them around, snap them to and into each other. You can also delete any blocks you don’t need.
Component Blocks
Each component in your app has its own set of blocks. You will click on the component to display the blocks you can then drag into the workspace.
EVENT HANDLERS
Event handler blocks in App Inventor are a gold color and are shaped as an open block, so you can snap blocks inside of it. Those blocks run only when that event happens.
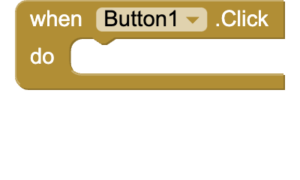
When Button is Clicked
You’ve already used this. When the user clicks a particular button, you would want the app to do something, like open another screen, or send a message.
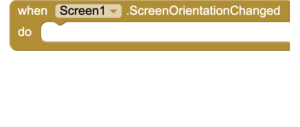
When Screen Orientation is Changed
If the user turns their phone from portrait (vertical) to landscape (horizontal), you might want to do something, like change the layout of the screen, or the size of components.

When Slider Position is Changed
A slider lets the user drag it to choose some value along it. This might trigger a change to another component. For example, you might increase the size the pen in a drawing app using a slider.
FUNCTIONS
Functions are blocks of code that do something. They can be run many times within an app. In App Inventor, functions blocks are colored purple. Some languages refer to functions as methods, or procedures. In fact, App Inventor generally refers to these as procedures. They do something, so you can think of them as action blocks.
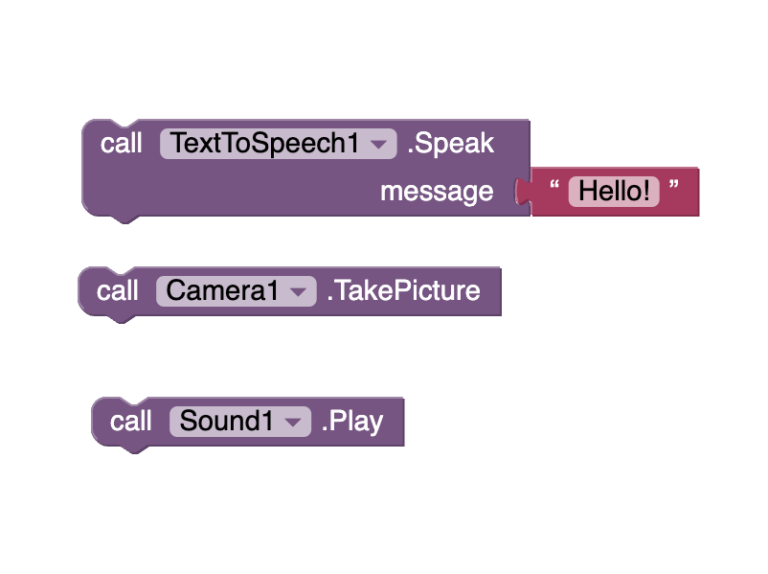
TextToSpeech.Speak
The TextToSpeech component can say or speak whatever text you want it to speak.
Camera.TakePicture
The Camera component can take a picture with the phone.
Sound.Play
The Sound component can play a sound.
SETTERS AND GETTERS
Green blocks relate to component’s and their properties.
The light green blocks that can snap to another block are called getters, because you are getting the value of the property.
Setters are darker green, and they can be snapped to, with an open slot on the end. This allows you to set the value of the property.
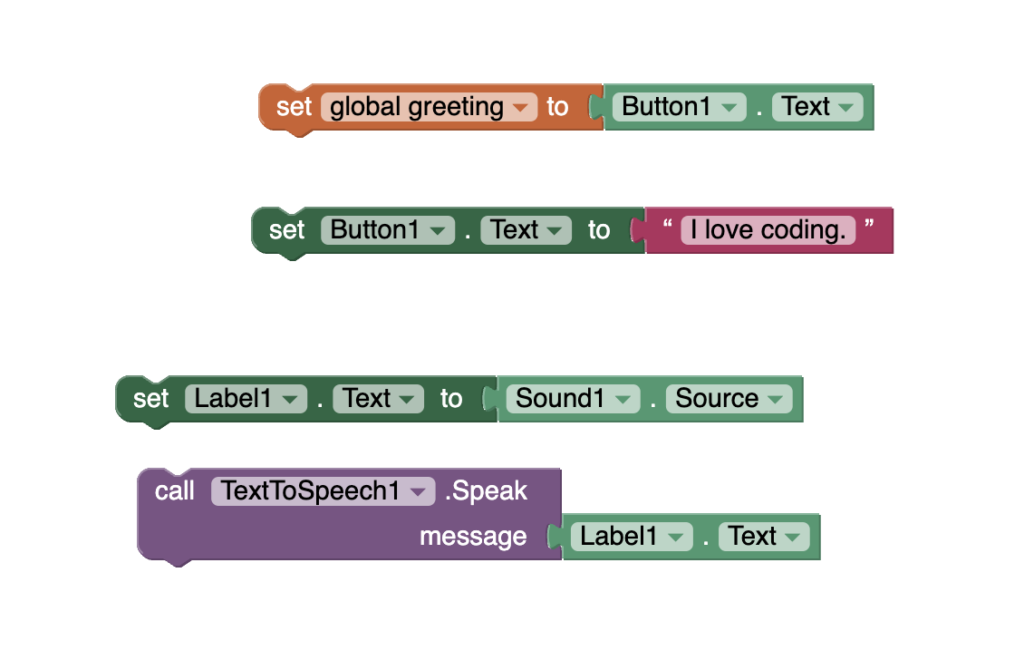
Text of Button1
The Text of Button1 is gotten and stored in the variable global greeting.
Text of Button1
The Text of Button1 is set to the words “I love coding. The words "I love coding" will appear on the button.
Text of Label1
The Text of Label1 is set to the Source of Sound1. This means the sound filename would be displayed in Label1.
Source of Sound1
Sound1's Source (the sound filename) is gotten and stored in Label1's Text property, Setting the text of a label essentially displays the value in the label, so the name of the sound file would appear in Label1.
Text of Label1
TextToSpeech gets the contents of Label1's Text and speaks it. Whatever text is displayed in Label1 would be spoken.
Mentor Tip
Best practices: Remind the students that this is exactly like what real coding is like. The weird vocabulary (functions, getters, setters) that we are using is exactly what coders use.
Guiding Questions to ask students: Can you think of some day-to-day functions you do? (example: baking a cake, brushing your teeth – anything that has repeatable steps). Functions are very similar to algorithms!
Mentor tips are provided by support from AmeriCorps.
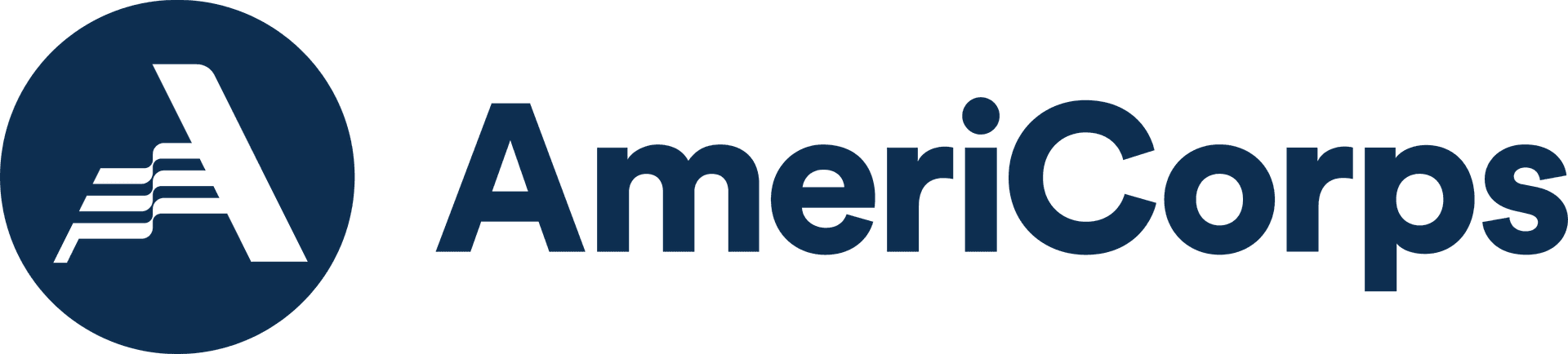
DATA BLOCKS
When you use setter blocks, you might use some other built-in blocks that represent data, or information that can be used in your app. Some examples of data blocks that can be used are seen below.
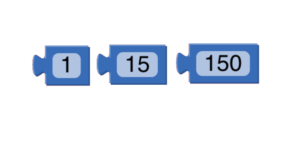
Numbers
Number values can be used as data in an app. These blocks can be found in the Math drawer in the blocks palette.
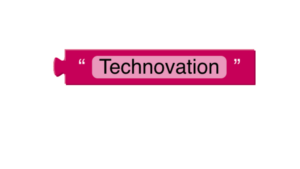
Text or Strings
Text, sometimes called strings, can be used as data. These are letters, words, and sentences that can be used in an app. The blocks are found in the Text drawer of the Blocks palette.
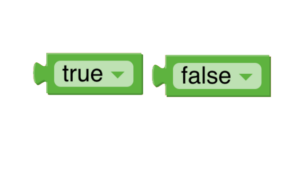
Boolean
This data type has only two possible values – true or false. These blocks can set or test the “state” of something and will be used in conditionals, which you will learn more about.
ACTIVITY: SOUNDBOARD TUTORIAL
Follow the video tutorial below
and follow Dave’s instructions in the video below.
Note that the video starts at 1:11 to skip over loading instructions. These instructions are not needed if you loaded the starter project using the link above.
CHALLENGE
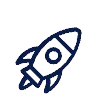
In the activity, you have used:
- Event Handler blocks
- Function blocks
Let’s try using some setter and getter blocks by adding to your Soundboard app.
When the user clicks on any picture to play the associated speech, change the background color of the screen.
You can change it to one particular color, but then you cannot easily change it back to the starting color, black, so instead, change the screen’s background to a random color.
Hint: Check the Color drawer and look for the make a color block. Then look in the Math drawer for a random integer block. Colors are made up of 3 numbers, RGB for red, green, blue, that range from 0-255.
REFLECTION
Now that you’ve learned a little bit more about the different code blocks, think about your app solution:
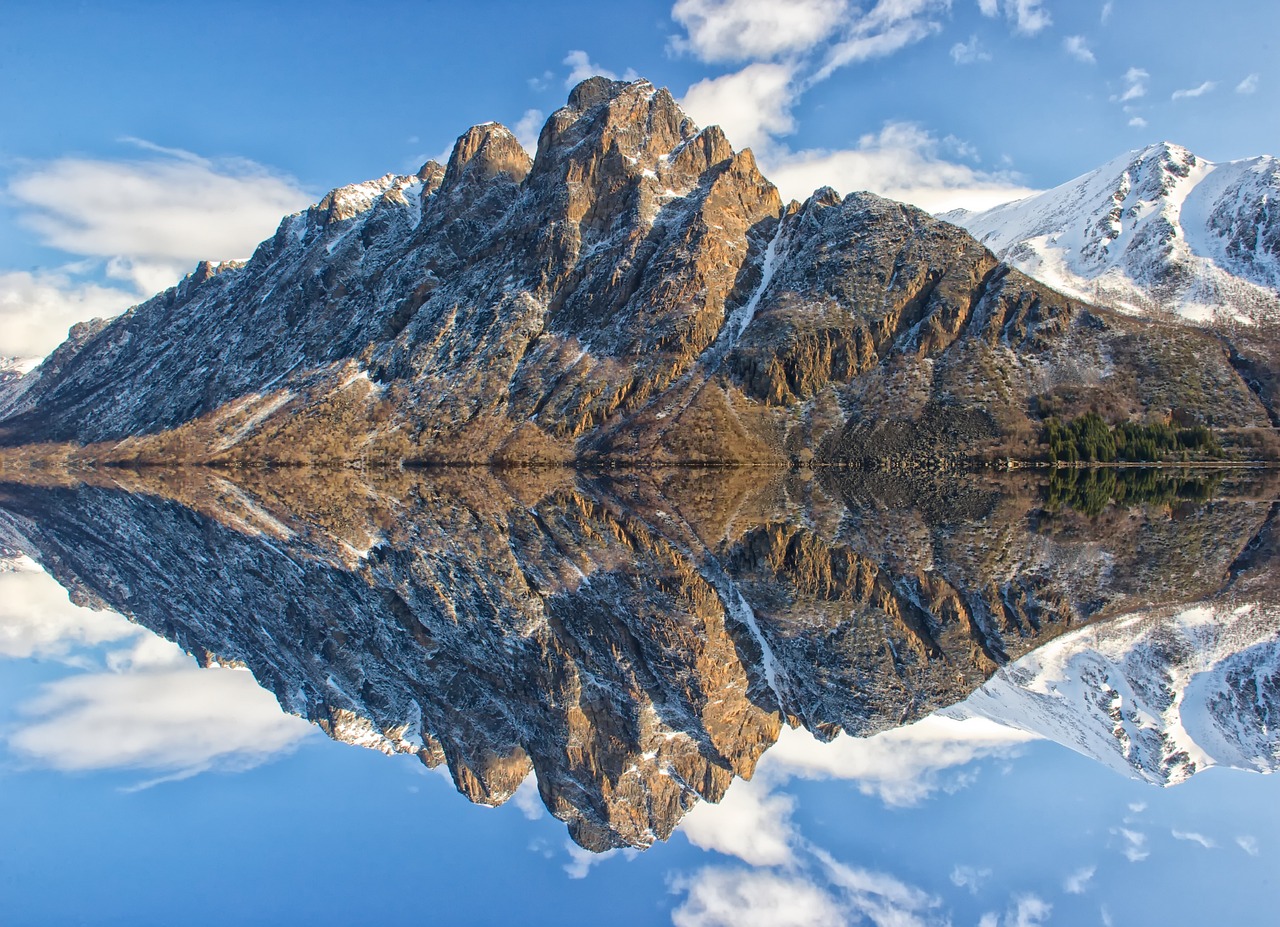
REVIEW OF KEY TERMS
- User Interface – everything in your app that a user can interact with
- Designer – window where you can add components to your app and design how they look
- Blocks Editor – window where you code the blocks for your app
- Event – something that happens to trigger code to run
- Event Handler– code blocks that tells your app what to do when an event happens
- Event Driven Programming – programming based on events, rather than running entire code from top to bottom
- Functions – a block of code that runs, and can be run multiple times
ADDITIONAL RESOURCES
Check out more of Dave Wolber’s App Inventor beginner tutorials at appinventor.org